Custom Handlebars Helpers for Ghost
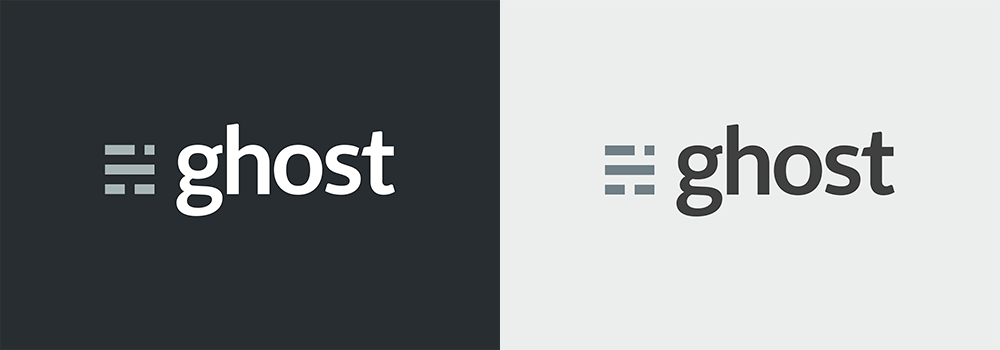
Plugins, or apps, are still in their infancy for the Ghost blogging platform, as such people have resorted to adding custom Handlebars helpers using a variety of hacks.
One of these is shown at https://apatchofcode.com/adding-custom-handlebars-for-ghost-equals-awesome/
Here is an alternative implementation that makes use of the fledgling Ghost Apps feature.
Installation
Create a new directory in contents/apps
eg:
mkdir contents/apps/myhelpers
Place package.json
and index.js
in the directory.
To enable the app you need to manually add it to the database as described at: https://github.com/TryGhost/Ghost/wiki/Apps-Getting-Started-for-Ghost-Devs
Restart ghost
Usage
Simply use the new helper in your template, eg:
{{#compare 'apples' '===' 'oranges'}}
<p>Totally not the case.<p>
{{/compare}}
{{#compare 'apples' 'typeof' 'string'}}
<p>I think we're onto something.<p>
{{/compare}}
package.json
{
"name":"my-helpers",
"version": "0.0.1",
"dependencies": {
"ghost-app": "0.0.2"
},
"ghost": {
"permissions": {
"helpers": ["compare"]
}
}
}
index.js
var App = require('ghost-app'),
myHelpers;
myHelpers = App.extend({
install: function() {},
uninstall: function() {},
activate: function() {
this.app.helpers.register('compare', this.compareHelper)
},
deactivate: function() {},
compareHelper: function (v1, operator, v2, options) {
switch (operator) {
case '==':
return (v1 == v2) ? options.fn(this) : options.inverse(this);
case '===':
return (v1 === v2) ? options.fn(this) : options.inverse(this);
case '!=':
return (v1 != v2) ? options.fn(this) : options.inverse(this);
case '!==':
return (v1 !== v2) ? options.fn(this) : options.inverse(this);
case '<':
return (v1 < v2) ? options.fn(this) : options.inverse(this);
case '<=':
return (v1 <= v2) ? options.fn(this) : options.inverse(this);
case '>':
return (v1 > v2) ? options.fn(this) : options.inverse(this);
case '>=':
return (v1 >= v2) ? options.fn(this) : options.inverse(this);
case '&&':
return (v1 && v2) ? options.fn(this) : options.inverse(this);
case '||':
return (v1 || v2) ? options.fn(this) : options.inverse(this);
case 'typeof':
return (typeof v1 == v2) ? options.fn(this) : options.inverse(this);
default:
return options.inverse(this);
}
}
});
module.exports = myHelpers;