2.2" Display for Raspberry Pi
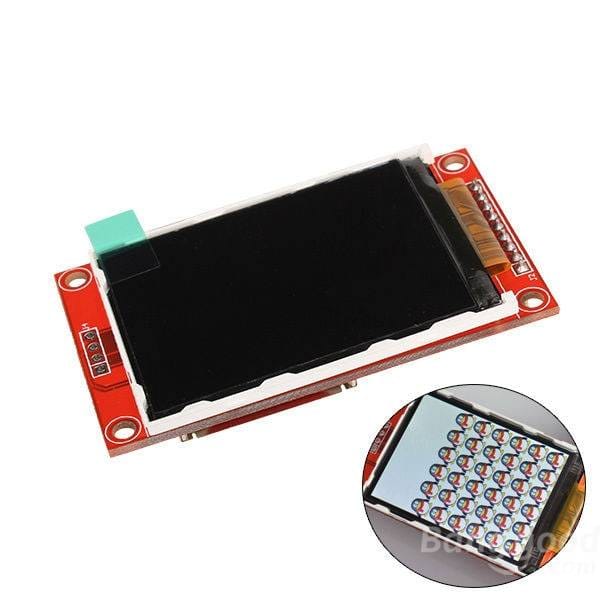
These cheap 2.2" LCDs are pretty handy for a Raspberry Pi.
Connect them like this:
Use Notoro's fbtft modules to get them working with Linux. They are included in the Raspberry Pi Raspbian image.
Edit /etc/modules and add (for landscape orientation):
fbtft_device name=adafruit22a rotate=90
Edit /boot/cmdline.txt to put login console on the LCD, add the following to the end:
fbcon=map:10
Edit /boot/config.txt and add or uncomment the following to enable SPI:
dtparam=spi=on
User mode access using PyGame and DirectFB took a bit of sleuthing, bit I finally nailed it down.
- Append the modes for the LCD to
/etc/fb.modes
fbset --info -fb /dev/fb1|sed -n '/mode/,/endmode/p'|sudo tee -a /etc/fb.modes
- Create
~/.directfbrc
with:
fbdev=/dev/fb1
quiet
no-vt
no-vt-switch
no-banner
mode=320x240
depth=16
- Check permissions and ownership of /dev/fb1
pi@raspberry ~ $ ls -l /dev/fb1
crw-rw---T 1 root video 29, 1 Jun 14 16:50 /dev/fb1
- Make your user is in the
video
group:
pi@raspberry ~ $ groups
pi adm dialout cdrom sudo audio video plugdev games users netdev gpio i2c spi input
- Use the following code to initialise your PyGame application
"""Ininitializes a new pygame screen using the framebuffer"""
# Based on "Python GUI in Linux frame buffer"
# http://www.karoltomala.com/blog/?p=679
disp_no = os.getenv("DISPLAY")
if disp_no:
print "I'm running under X display = {0}".format(disp_no)
# Check which frame buffer drivers are available
# Start with fbcon since directfb hangs with composite output
drivers = ['fbcon', 'directfb', 'svgalib']
found = False
for driver in drivers:
# Make sure that SDL_VIDEODRIVER is set
if not os.getenv('SDL_VIDEODRIVER'):
os.putenv('SDL_VIDEODRIVER', driver)
try:
pygame.display.init()
except pygame.error:
print 'Driver: {0} failed.'.format(driver)
continue
found = True
break
if not found:
raise Exception('No suitable video driver found!')
size = (pygame.display.Info().current_w, pygame.display.Info().current_h)
print "%s: framebuffer size: %d x %d" % (driver, size[0], size[1])
screen = pygame.display.set_mode(size, pygame.FULLSCREEN)